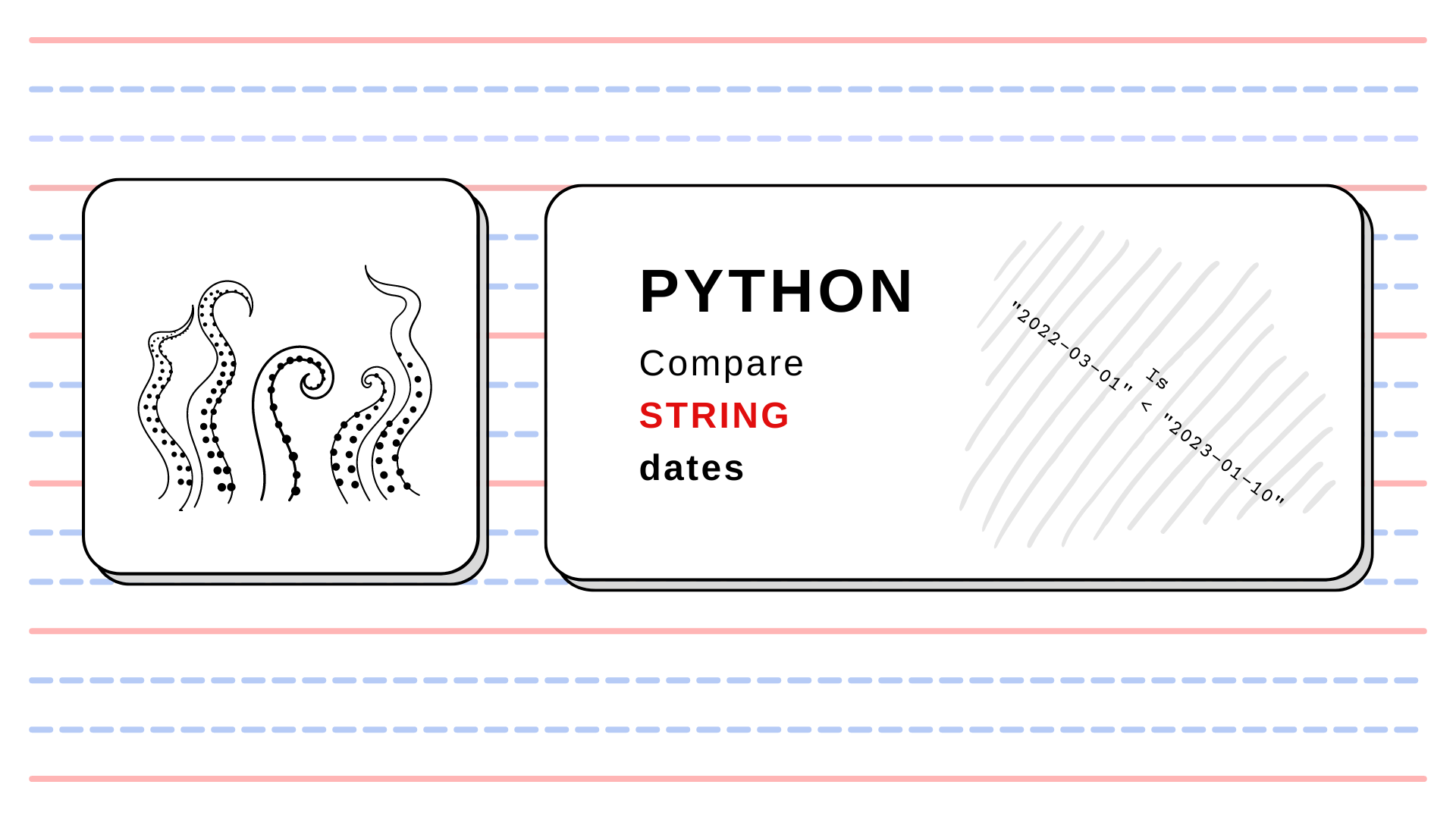
Toolbox: Compare dates as string in Python
January 1, 2023
Have you encountered a situation when you must compare two dates written as strings? For example:
"2022-01-01" < "2022-01-02"
This is a common problem with web and mobile app development, where dates are stored as strings. The best example of this behavior is Amazon’s DynamoDB. How to compare dates as strings? It can be done with a relatively simple function:
from datetime import datetime def compare_str_dates(past_time: str, future_time: str, length=10, format='%Y-%m-%d') -> bool: """ Function compares two dates written as strings. Parameters ---------- past_time : str An expected past date as a string. future_time : str An expected future date as a string. length : int, default=10 The length of a string with date characters. format : str, default='%Y-%m-%d' The format of a date. Returns ------- : bool ``True`` if the future date is in future in comparison to the past date. """ past_time = past_time[:length] future_time = future_time[:length] past = datetime.strptime(past_time, format) future = datetime.strptime(future_time, format) if future > past: return True return False
An example:
PAST = "2022-01-01" FUTURE = "2022-01-02 22:01:00" comparison = compare_str_dates(PAST, FUTURE) print(comparison)
>> True
Subscribe
Login
0 Comments